A first look at Ruby classes
You got class or you don't
July 14th, 2015
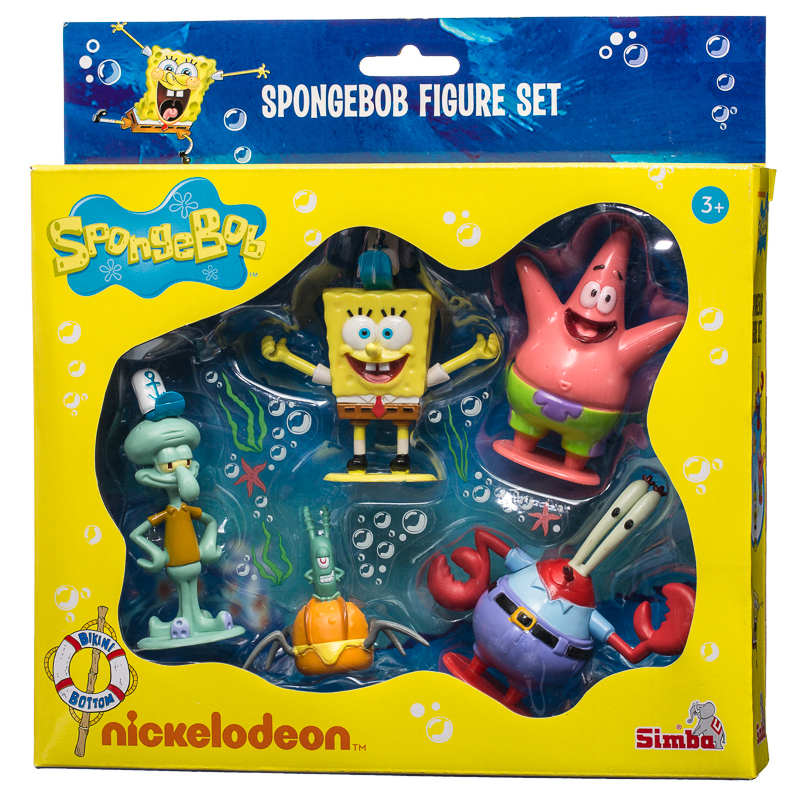
Week-5 at Dev Bootcamp is our third week studying Ruby, focusing on classes. To me, understanding the concept of classes is also grasping the whole potential of Ruby as a language to build applications. As you know, Ruby is object-oriented, which means that everything you work with is an object with specific properties and behaviours, like in real life an object can be a car, a book or even a SpongeBob figure. Objects sharing the same properties and behaviours are grouped under the same class of objects. In other words, you can say that an object from a specific class is an instance of that class.
To drive the point home here is how we would work with the SpongeBob figure set class
class Spongebob_figureset def initialize(character_name, specie, role, key_quote) @character_name = character name @specie = specie @role = role @key_quote = key_quote end def print puts @character_name puts @specie puts @role puts @key_quote end
In this code we have initialized a new class called Spongebob_figureset, a class always start with the initialize method, which sets the properties of an object of that class, also called instance of the class. In short, properties spell the defining traits of an instance, or what it takes to create a fully capable object belonging to the class. In this example an object is created using four arguments: character_name, specie, role, key_quote.
After the initialize method, we can declare other methods that will define behaviours specific to the class, these methods are called class method or instance method. Above, the second instance method we define is print. When this method is called on an instance of the Spongebob_figureset Class, it prints the figure info to the console, as illustrated below.
squidward = Spongebob_figureset.new("Squidward Q. Tentacles", "Octopus", "cashier of the Krusty Krab", "No, Patrick, mayonnaise is not an instrument.") squidward.print Squidward Q. Tentacles Octopus cashier of the Krusty Krab No, Patrick, mayonnaise is not an instrument.
We could have developed a whole suite of methods to obtain specific behaviours for the class. That’s how we can start grasping the concept of classes for applications. Let’s move to another example: an application like Groupon, which was developed in Ruby, could create a Class named deals, that would be initialized with arguments like category, provider, discount, #deals_available. Whenever the number of deals available needs to be updated, we could write a specific method that will destructively change the variable (i.e. change it permanently).
I hope this was helpful! As usual, I would love to get in touch, use the icons below!